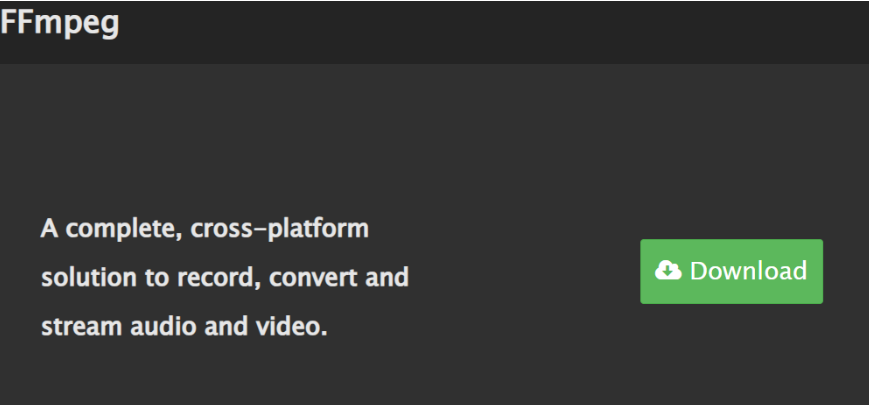
If you’re working with large video files and need to reduce their size while maintaining acceptable quality, FFmpeg provides a powerful suite of tools for video compression. In this guide, we’ll show you how to reduce video size using FFmpeg in combination with JavaScript (using the fluent-ffmpeg
package), and we’ll explain key concepts like video resolution, bitrate, encoding/decoding, and codecs that influence video size and quality.
Key Concepts: Video Resolution, Bitrate, Encoding/Decoding, and Codecs
To understand how to reduce video size effectively, you first need to grasp the key concepts that control video quality and file size. These are video resolution, bitrate, encoding/decoding, and codecs.
1. Video Resolution
Video resolution refers to the dimensions of the video in terms of width and height (measured in pixels). For example, a video with a resolution of 1920x1080
(Full HD) means the video is 1920 pixels wide and 1080 pixels tall.
- Higher resolution means more pixels, which results in better image quality but increases the file size.
- Lower resolution reduces the number of pixels, which decreases the file size but also reduces video quality.
Common video resolutions include:
- 720p (HD): 1280×720 pixels
- 1080p (Full HD): 1920×1080 pixels
- 1440p (2K): 2560×1440 pixels
- 2160p (4K): 3840×2160 pixels
You can resize the video resolution to reduce its file size without affecting other properties of the video too much.
2. Bitrate
Bitrate refers to the amount of data processed per unit of time in a video. It’s typically measured in kilobits per second (kbps) or megabits per second (Mbps).
- Higher bitrate means more data is used to represent the video, which improves video quality but increases the file size.
- Lower bitrate uses less data, which reduces both the quality and file size.
For example, a video with a bitrate of 2000 kbps will generally offer better quality than a video with a bitrate of 500 kbps, but the file size will also be larger. Lowering the bitrate is a common technique for reducing file size.
3. Encoding and Decoding
Encoding is the process of compressing and converting raw video/audio data into a specific format, like MP4, using a codec. On the other hand, decoding is the reverse process of converting compressed data back to a usable format for playback.
- Video Encoding: The video is compressed using a codec (e.g., H.264, H.265).
- Audio Encoding: The audio is compressed using a codec (e.g., AAC, MP3).
The codec you choose, as well as the encoding settings (like bitrate, resolution, or compression level), directly affects the file size and quality of the output video.
4. What is a Codec and Why is it Important?
A codec (short for compressor-decompressor) is a software or algorithm used to compress (encode) and decompress (decode) digital media. Codecs are essential because they determine how efficiently a video or audio file is compressed, which in turn impacts the quality, file size, and compatibility of the file.
- Compression: Codecs compress the raw video and audio data to make files smaller, which makes it easier to store or transmit.
- Decompression: When the file is played, the codec decompresses the data and turns it into a format that can be displayed or played.
For example:
- Video codecs: H.264, H.265 (HEVC), VP9
- Audio codecs: AAC, MP3, Opus
The choice of codec impacts both video quality and compression efficiency:
- H.264 is a very common video codec that balances good compression with excellent compatibility and is widely supported across devices and platforms.
- H.265 (HEVC) is a more efficient codec, providing better compression (smaller files) at the same quality level, but it is not as universally supported as H.264.
- VP9 is an alternative to H.265, often used by YouTube and other Google platforms, offering high compression efficiency for web videos.
Similarly, AAC is a popular audio codec that provides high-quality sound at low bitrates, while MP3 is one of the oldest and most commonly used audio codecs.
Importance of Codecs:
- File Size and Quality: Some codecs offer better compression without sacrificing quality, making them ideal for reducing video file sizes while maintaining quality. For example, H.265 provides better compression than H.264.
- Compatibility: Not all devices support all codecs. For example, H.265 might not be as widely supported as H.264 on older devices or browsers.
- Streaming: Efficient codecs like H.265 and VP9 are important for streaming, as they allow better compression and faster loading times.
Choosing the right codec and encoding settings is essential to get the best balance between video quality, file size, and compatibility.
Step-by-Step Guide to Reducing Video Size Using FFmpeg and JavaScript
To reduce the size of a video using FFmpeg and JavaScript, we can use the fluent-ffmpeg
package. Follow these steps to perform basic video size reduction tasks.
Want to know how the performance increased by 94x for ffmpeg, read this
1. Install FFmpeg on Your System
Before using FFmpeg, make sure it is installed on your machine.
- macOS: Use Homebrew:
brew install ffmpeg
- Ubuntu/Debian: Use apt:
sudo apt install ffmpeg
- Windows: Download the FFmpeg binaries from the FFmpeg website and add them to your system’s PATH.
2. Install the fluent-ffmpeg
Node.js Package
Next, install the fluent-ffmpeg
package using npm (Node’s package manager):
npm install fluent-ffmpeg
3. Example 1: Reduce Video Resolution and Bitrate
One of the simplest ways to reduce video size is to resize the video resolution and reduce the video bitrate. Here’s how to do it in JavaScript:
const ffmpeg = require('fluent-ffmpeg');
// Input and output video paths
const inputVideo = 'input-video.mp4';
const outputVideo = 'output-video.mp4';
ffmpeg(inputVideo)
.output(outputVideo)
.videoCodec('libx264') // H.264 codec for video
.audioCodec('aac') // AAC codec for audio
.size('640x360') // Resize the video to 640x360 resolution
.audioBitrate('128k') // Set audio bitrate to 128 kbps
.videoBitrate('500k') // Set video bitrate to 500 kbps (adjust for file size)
.on('end', () => {
console.log('Video size reduced and saved as:', outputVideo);
})
.on('error', (err) => {
console.log('Error:', err.message);
})
.run();
In this example:
- Resolution is reduced to
640x360
, which decreases the number of pixels and reduces the file size. - Bitrate for video is set to
500k
, which reduces the amount of data used to represent the video, further reducing file size. - Audio bitrate is set to
128k
, which is a common setting for decent audio quality.
4. Example 2: Reducing Video Size by Lowering Bitrate
You can also reduce file size by lowering the video bitrate without changing the resolution.
ffmpeg('input-video.mp4')
.output('output-video.mp4')
.videoCodec('libx264') // H.264 codec for video
.audioCodec('aac') // AAC codec for audio
.videoBitrate('500k') // Lower the video bitrate to reduce file size
.audioBitrate('128k') // Lower the audio bitrate
.on('end', () => {
console.log('Video file size reduced successfully');
})
.on('error', (err) => {
console.log('Error:', err.message);
})
.run();
5. Example 3: Optimize Compression with CRF and Preset Options
FFmpeg allows you to control the quality and compression of the video using CRF (Constant Rate Factor) and preset options.
- CRF: Controls the quality of the encoding (lower CRF = better quality, higher CRF = more compression, lower quality).
- Preset: Controls the speed of encoding (slower presets result in better compression and smaller file size, but take more time to process).
“`javascript
ffmpeg(‘input-video.mp4’)
.output(‘output-video.mp4’)
.videoCodec(‘libx264’) // Use H.264 codec
.audioCodec(‘aac’) // Use AAC codec
.
Want to know how the performance increased by 94x for ffmpeg, read this