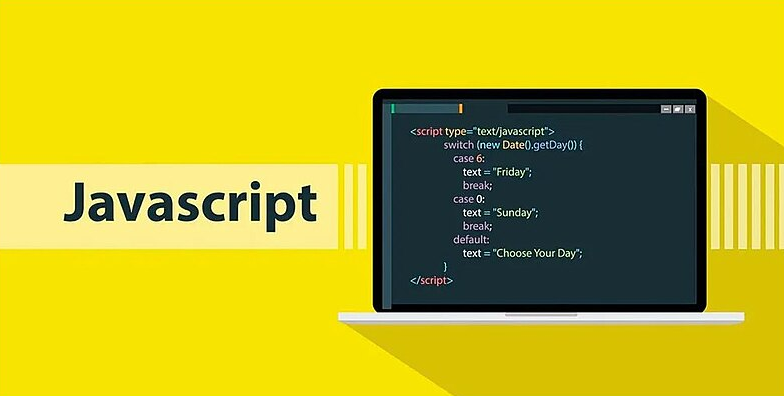
Explain closure in javascript with example:
Imagine You Have a Cookie Jar
Let’s start with something you already know. Imagine you have a cookie jar at home. You start with a certain number of cookies, say 10 cookies. Every time you take a cookie, the number of cookies in the jar decreases. So, if you take 1 cookie, now there are 9 cookies left.
Now, let’s say every time you take a cookie, you want the cookie jar to remember how many cookies are left, and tell you. So if you eat one cookie, it should say “You ate a cookie. Now there are 9 cookies left.” Then, if you eat another cookie, it says, “You ate a cookie. Now there are 8 cookies left.”
But here’s the cool part: even if you leave the room, the jar remembers how many cookies are left! Every time you come back to the jar, it still remembers how many cookies are there and can keep counting as you eat them.
The Cookie Jar in Programming
Now, in programming, we can do something similar to the cookie jar using functions. A function is like a set of instructions or a recipe for something, and just like how the cookie jar can remember how many cookies are left, a function can remember certain things, even after it has finished.
This special ability of a function to remember things is called a closure. It’s like the function keeps a little notebook (or memory) inside that it can use later.
Let’s see how we can create a cookie jar in JavaScript:
JavaScript Example: The Cookie Jar
1 2 3 4 5 6 7 8 9 10 11 12 | function cookieJar(initialCookies) { let cookies = initialCookies; // Start with a certain number of cookies return function() { cookies--; // Every time this function is called, take one cookie out console.log("You ate a cookie. Now there are " + cookies + " cookies left!"); }; } const myJar = cookieJar(10); // Start with 10 cookies in the jar myJar(); // "You ate a cookie. Now there are 9 cookies left!" myJar(); // "You ate a cookie. Now there are 8 cookies left!" myJar(); // "You ate a cookie. Now there are 7 cookies left!" |
Let’s Break It Down Step-by-Step:
- The
cookieJar
Function:
- This is like creating a cookie jar. The
cookieJar
function takes in the number of cookies you want to start with, and it remembers that number using a special variable calledcookies
.
2.The Inner Function:
- Inside the
cookieJar
function, there is another function (the one that counts the cookies). This inner function is like the part of the jar that remembers how many cookies are left. Every time you call it (like eating a cookie), it remembers and reduces the number of cookies by 1.
3. Returning the Inner Function:
- When you call
cookieJar(10)
, it returns the inner function. This means that even aftercookieJar
is finished, the inner function can still remember the number of cookies. So you can callmyJar()
multiple times, and it will continue to track how many cookies are left because of the closure.
What is Happening Behind the Scenes?
- The inner function,
myJar
, remembers the value ofcookies
(how many cookies are in the jar), even though the outer function (cookieJar
) has already finished. - This is possible because of the closure: the inner function “closes over” the variable
cookies
, keeping track of it even when it’s called later.
Why is This So Cool?
So, what makes this special?
Normally, when a function finishes, it forgets everything it did. But with a closure, the function remembers important things (like the number of cookies), even after it’s done. This is very useful in programming because we can make programs that keep track of things over time, without needing to start from scratch each time.
For example:
- You could use closures to keep track of a score in a game.
- Or, you could create a counter that counts how many times something happens, and the counter will keep remembering its number, even if you leave and come back later.
Closure in JavaScript: A Quick Recap
- Closure is when a function remembers things even after it has finished running.
- It allows the inner function to keep using data (like the number of cookies) even after the outer function is done.
- In JavaScript, closures help us save important information inside functions, so we can use it later.