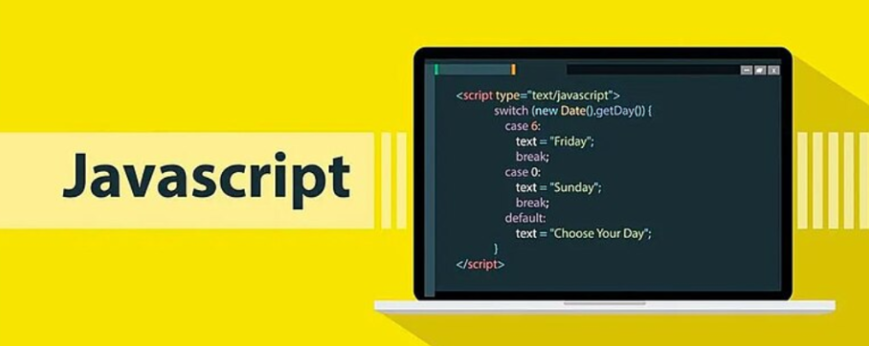
5 javascript concepts every beginner should know
Alright, imagine JavaScript is like your super cool, magical robot that helps you do things on the computer. Now, just like how you use different tools for different tasks, JavaScript has some special tools called map, reduce, filter, find, and forEach. These tools help you work with lists (arrays) of things, like your favorite candies, toys, or even homework assignments. Let’s break these tools down in a fun way that even a 5th grader can understand!
1. Map: The Candy Wrapper Machine
Imagine you have a huge pile of candies (an array). But you want to wrap every candy in a new, sparkly wrapper. You don’t need to unwrap each candy individually – you just tell the robot to “map” over the pile and wrap them all with the same shiny wrapper.
In JavaScript, the map tool does exactly that. It takes every item from the list, does something to it (like wrapping it), and gives you a brand new list with all the updated items.
Here’s how it works:
let candies = [1, 2, 3, 4];
let wrappedCandies = candies.map(candy => candy * 2); // We wrap the candies by doubling them!
console.log(wrappedCandies); // [2, 4, 6, 8]
It doubled every candy, like making them extra sweet!
2. Reduce: The Super Sweeper
Let’s say you’ve got a huge mess of toys (or any numbers), and you want to sweep them into one big toy box. The reduce tool is like your superhero broom that picks up everything in the array and smooshes it into a single thing, like a giant toy box.
So, if you have a bunch of numbers and you want to add them all together, reduce can do it for you:
let numbers = [1, 2, 3, 4];
let total = numbers.reduce((acc, num) => acc + num, 0); // We’re sweeping up all the numbers into one pile!
console.log(total); // 10
It added all the numbers together like magic, and now you have one giant number!
3. Filter: The Candy Sorter
Now, imagine you have a big bag of candies, but some of them are spicy and you don’t like them. The filter tool is like a superpower that lets you sort out all the candies you don’t want and only keep the ones you like!
Here’s how filter works:
let candies = ['chocolate', 'spicy', 'caramel', 'spicy'];
let goodCandies = candies.filter(candy => candy !== 'spicy'); // We’re filtering out the spicy ones!
console.log(goodCandies); // ['chocolate', 'caramel']
Now, you only have the candies you love, no spicy stuff!
4. Find: The Lost Toy Finder
Okay, let’s say you’ve lost your favorite toy in a huge toy box, and you need to find it fast! The find tool is like a super detective that looks through all the toys and picks out the first one that matches your description.
So, if you want to find the first “red” toy, here’s how you can do it:
let toys = ['blue car', 'red ball', 'green dinosaur', 'red kite'];
let redToy = toys.find(toy => toy.includes('red')); // Searching for the first red toy
console.log(redToy); // 'red ball'
The robot found the first “red” toy! Super detective skills!
5. ForEach: The Toy Organizer
Now, imagine you want to organize all your toys one by one. ForEach is like a super helper robot that just goes through each toy, one at a time, and does something to it, like lining them up, dusting them off, or giving them a sticker.
For example, if you want to say “hello” to every toy in your toy box:
let toys = ['ball', 'teddy bear', 'robot'];
toys.forEach(toy => console.log(`Hello, ${toy}!`)); // Saying hello to each toy
Closures in javascript
Javascript objects