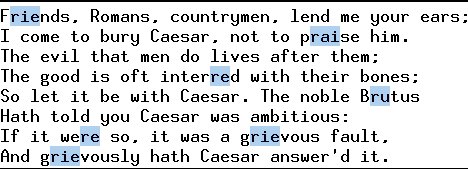
Regex in javascript made simpler, we will first cover basic symbols, like forward slashes (/
), and other symbols and brackets in regex that help define different kinds of patterns. Let’s break it all down in a simple way.
1. Forward Slashes (/
)
We already discussed that forward slashes (/
) are like the “box” that wraps the entire regex pattern. They don’t change what the pattern does; they just mark the beginning and end of it.
- Example:
/pattern/
/
= Marks the beginning and end of the regex pattern.- The part inside (e.g.,
pattern
) is what you’re actually looking for.
2. Square Brackets ([]
)
Square brackets [ ]
are used to define a set of characters that you want to match. Inside the brackets, you can list the characters or numbers you’re looking for.
Explanation:
- The square brackets
[ ]
tell the regex to match one character from the set of characters inside them. - It means “any one of these characters”.
Example 1: Matching a Single Character
If you want to find a vowel (like a
, e
, i
, o
, u
), you can use:
/[aeiou]/
[aeiou]
means: match any one of these letters (eithera
,e
,i
,o
, oru
).- This pattern will find any vowel in a string.
Example 2: Matching Digits
If you want to match a single digit (any number between 0 and 9), you can use:
/\d/
\d
inside the square brackets means any digit (0-9).- You could also use
[0-9]
, which is exactly the same as\d
.
Example 3: Matching Letters (Uppercase or Lowercase)
If you want to match any letter, either uppercase or lowercase, you can use:
/[a-zA-Z]/
[a-zA-Z]
means: match any lowercase or uppercase letter.
3. Parentheses (()
)
Parentheses ( )
are used for grouping parts of your regex pattern. They help you organize and apply other regex operations to a group of characters.
- Example:
/abc/
- This just matches the characters “abc” in order.
But with parentheses, you can group things together. For example:
/(abc)+/
(abc)
groups the charactersa
,b
, andc
together.- The
+
outside means “one or more” of the group. So this matches “abc”, “abcabc”, “abcabcabc”, etc.
Example 2: Grouping Numbers and Letters
If you want to find numbers followed by letters, you can use:
/(\d+)[a-zA-Z]+/
(\d+)
groups one or more digits together.[a-zA-Z]+
matches one or more letters.
4. Curly Braces ({}
)
Curly braces {}
are used to specify the exact number of times something should appear. This is useful when you want to match a specific quantity of characters, digits, etc.
Example 1: Matching Exactly 3 Digits
If you want to match exactly 3 digits (like 123
), you use:
/\d{3}/
\d{3}
means exactly 3 digits.
Example 2: Matching a Range of Characters
If you want to match a range of characters, like 2 to 4 digits, you can use:
/\d{2,4}/
\d{2,4}
means 2, 3, or 4 digits.
5. Square Brackets ([]
) vs. Parentheses (()
)
- Square Brackets (
[ ]
) are used for matching one of several characters from the group. It’s like saying, “pick one”. - Example:
/[abc]/
matches eithera
,b
, orc
. - Parentheses (
( )
) are used for grouping things together, so you can apply actions (like+
or*
) to a group of characters. - Example:
/(abc)+/
matches one or more occurrences of “abc”.
6. Other Important Symbols
Here are a couple of other useful symbols you’ll often see in regular expressions:
.
(Dot): The dot matches any single character (except for line breaks).- Example:
/a.c/
will matchabc
,axc
,a1c
, but notac
. +
(Plus): This means one or more of the previous character or group.- Example:
/\d+/
will match1
,123
,99999
, but not empty. *
(Star): This means zero or more of the previous character or group.- Example:
/\d*/
will match1
,123
,99999
, or even an empty string (nothing). ?
(Question Mark): This means the previous character or group is optional (it can appear zero or once).- Example:
/a?b/
will matchb
orab
.
Putting It All Together with Examples
Let’s use these symbols to create regex patterns for names, numbers, and emails.
1. Finding Names:
We want to find names that start with a capital letter and then have lowercase letters.
Regex: /[A-Z][a-z]+/
[A-Z]
means: any capital letter at the start.[a-z]+
means: one or more lowercase letters after the capital letter.
Example:
let text = "John and Alice went to the park.";
let regex = /[A-Z][a-z]+/g;
let names = text.match(regex);
console.log(names); // Output: ['John', 'Alice']
2. Finding Numbers:
If you want to match numbers (whether they are whole numbers or decimals), you can use:
Regex: /\d+/
\d
matches any digit (0-9).+
means one or more digits.
Example:
let text = "I have 5 apples and my brother has 10.5 oranges.";
let regex = /\d+/g;
let numbers = text.match(regex);
console.log(numbers); // Output: ['5', '10', '5']
3. Validating Email Addresses:
An email address follows a pattern: it has a username, the @
symbol, and a domain.
Regex: /^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$/
^[a-zA-Z0-9._%+-]+
: The username before the@
, which can include letters (both uppercase and lowercase), numbers, and some special characters.@[a-zA-Z0-9.-]+
: The domain part after the@
, which can include letters, numbers, dots (.
), and dashes (-
).\.[a-zA-Z]{2,}$
: The top-level domain (TLD) (like.com
,.org
), which must be at least 2 characters long.
Example:
let email = "[email protected]";
let regex = /^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$/;
if (email.match(regex)) {
console.log("Valid email address!");
} else {
console.log("Invalid email address!");
}
Summary of Key Brackets and Symbols:
[]
: Square brackets to match one character from a list (like vowels or digits).()
: Parentheses to group characters together, often with a+
or*
applied to the group.{}
: Curly braces to match exact numbers of characters..
: Dot matches any character (except line breaks).+
,*
,?
: Symbols that apply to a character or group to specify how many times it should appear.
I hope this helps! Regular expressions are like magic wands for searching and finding things
You may also like:
Closures in javascript
Javascript objects