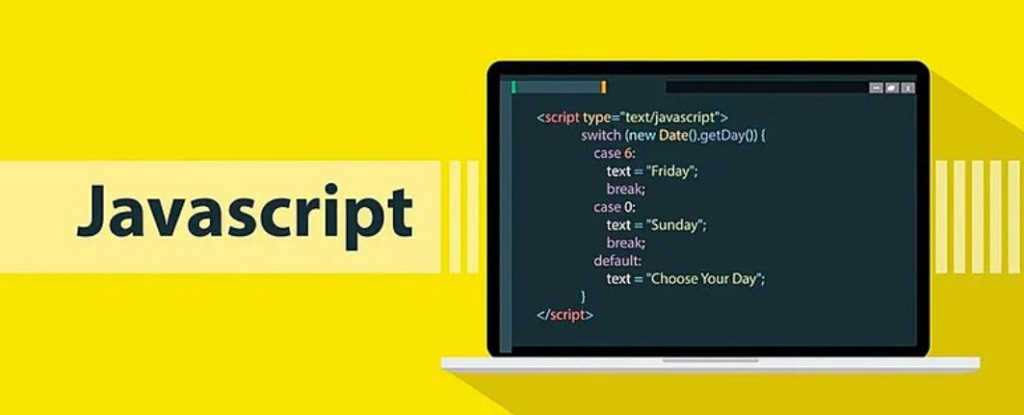
Explain javascript objects in simple way
JavaScript objects are like special toy boxes, and inside these boxes, you can do a lot of interesting things. Now that you understand the basics of objects, let’s explore some powerful tools you can use to work with these toy boxes. These tools help you copy, compare, inspect, create new boxes, and even get all the toys inside them.
Let’s dive into these important object methods that will help you control and manipulate objects like a pro!
1. Object.assign(): Copying Objects
Imagine you have a toy box, and you want to make a copy of it, so you don’t change the original one. You can use Object.assign()
to copy the properties from one object (toy box) to another.
Example:
let toyBox1 = {
toys: ["teddy bear", "lego set"],
color: "red",
};
let toyBox2 = Object.assign({}, toyBox1); // Copy toyBox1 into toyBox2
toyBox2.color = "blue"; // Changing color in toyBox2 doesn't affect toyBox1
console.log(toyBox1.color); // Prints: red
console.log(toyBox2.color); // Prints: blue
Explanation:
Object.assign()
creates a shallow copy oftoyBox1
intotoyBox2
. This means if there are objects or arrays inside, they will still point to the same thing, but for simple properties likecolor
, they are copied directly.
2. Object.getOwnPropertyNames(): Get All Property Names
If you want to find out all the toys (properties) inside your toy box, you can use Object.getOwnPropertyNames()
to get a list of all the keys (names) of the properties.
Example:
let toyBox = {
toys: ["teddy bear", "lego set"],
color: "red",
owner: "Alice"
};
let propertyNames = Object.getOwnPropertyNames(toyBox);
console.log(propertyNames); // Prints: ['toys', 'color', 'owner']
Explanation:
Object.getOwnPropertyNames()
returns an array of property names inside thetoyBox
object.
3. Object.getOwnPropertyDescriptors(): Inspect Object Properties
If you want to know detailed information about each property in your toy box, including whether it’s writable, enumerable, or configurable, you can use Object.getOwnPropertyDescriptors()
.
Example:
let toyBox = {
toys: ["teddy bear", "lego set"]
};
let descriptors = Object.getOwnPropertyDescriptors(toyBox);
console.log(descriptors.toys);
// Prints: { value: [ 'teddy bear', 'lego set' ], writable: true, enumerable: true, configurable: true }
Explanation:
Object.getOwnPropertyDescriptors()
gives detailed info about each property. For example, it tells you if you can change the toys in the toy box (writable: true
), if the property shows up when you look through the object (enumerable: true
), and if the property can be deleted (configurable: true
).
4. Object.is(): Comparing Two Objects
Want to compare two toy boxes and see if they are exactly the same, not just by reference, but also by their value? Use Object.is()
to do that.
Example:
let toyBox1 = { color: "red" };
let toyBox2 = { color: "red" };
console.log(Object.is(toyBox1, toyBox2)); // Prints: false
Explanation:
Object.is()
checks if both objects are exactly the same. In this case, even though both objects have the samecolor
, they are different objects in memory, soObject.is()
returnsfalse
.
5. Object.create(): Creating New Toy Boxes
If you want to create a new toy box with some default toys inside, and you also want this new box to have certain properties from another toy box, you can use Object.create()
.
Example:
let toyBoxPrototype = {
color: "red",
toys: ["teddy bear", "lego set"]
};
let newToyBox = Object.create(toyBoxPrototype);
newToyBox.owner = "Bob";
console.log(newToyBox.color); // Prints: red
console.log(newToyBox.toys); // Prints: ['teddy bear', 'lego set']
console.log(newToyBox.owner); // Prints: Bob
Explanation:
Object.create()
creates a new object that inherits properties from another object (thetoyBoxPrototype
in this case).- You can still add new properties to the new object (like
owner
).
6. Object.keys(): Get All Keys (Properties) of the Object
If you want to know the names of all the toys in the toy box (the keys of the object), you can use Object.keys()
.
Example:
let toyBox = {
toys: ["teddy bear", "lego set"],
color: "red",
};
let keys = Object.keys(toyBox);
console.log(keys); // Prints: ['toys', 'color']
Explanation:
Object.keys()
returns an array of keys of the object, which are the names of the properties.
7. Object.values(): Get All Values of the Object
Want to know what’s inside the toy box without knowing the names of the toys? You can use Object.values()
to get the values of the properties.
Example:
let toyBox = {
toys: ["teddy bear", "lego set"],
color: "red",
};
let values = Object.values(toyBox);
console.log(values); // Prints: [['teddy bear', 'lego set'], 'red']
Explanation:
Object.values()
gives you the values of the properties inside the object (in this case, the toys and the color).
8. Object.entries(): Get Both Keys and Values
If you want both the name and the value of each property in your toy box, use Object.entries()
. It will give you an array of arrays, where each inner array contains a key and its value.
Example:
let toyBox = {
toys: ["teddy bear", "lego set"],
color: "red",
};
let entries = Object.entries(toyBox);
console.log(entries); // Prints: [['toys', ['teddy bear', 'lego set']], ['color', 'red']]
Explanation:
Object.entries()
returns an array of arrays, where each array has two elements: the key and the value.
9. Object.getOwnPropertyDescriptor(): Detailed Property Info
This method gives you detailed information about a specific property in your object, like whether you can change it, whether it’s enumerable (can be listed), or configurable (can be deleted).
Example:
let toyBox = {
toys: ["teddy bear", "lego set"],
};
let toyDescriptor = Object.getOwnPropertyDescriptor(toyBox, "toys");
console.log(toyDescriptor);
// Prints: { value: [ 'teddy bear', 'lego set' ], writable: true, enumerable: true, configurable: true }
Explanation:
Object.getOwnPropertyDescriptor()
gives you a descriptor for a property (in this case,toys
), telling you if it can be modified, listed, or deleted.
Conclusion
Now you know some amazing techniques for working with JavaScript objects, like:
- Manipulating objects using
Object.assign()
,Object.create()
, andObject.keys()
. - Comparing objects with
Object.is()
andObject.entries()
. - Controlling and inspecting object properties using methods like
Object.getOwnPropertyDescriptors()
,Object.getOwnPropertyNames()
, and more!