Introduction
JavaScript, the cornerstone of modern web development, empowers developers to create dynamic and interactive web applications. At the heart of many web applications lie CRUD operations—Create, Read, Update, and Delete—essential for managing data. In this comprehensive tutorial, we will explore how to implement CRUD operations in JavaScript, accompanied by detailed code examples and corresponding HTML forms for practical application.
Understanding JavaScript CRUD Operations form the backbone of data management in web applications:
- Create: Adding new data entries to a system.
- Read: Retrieving existing data entries from the system.
- Update: Modifying existing data entries.
- Delete: Removing data entries from the system.
These operations are fundamental for maintaining data integrity and enabling user interaction in web applications.
Setting Up the Environment Before we delve into implementation, ensure you have a text editor and a web browser. You can use popular editors like Visual Studio Code, Sublime Text, or Atom. A basic understanding of HTML, CSS, and JavaScript is recommended.
/ JavaScript code for CRUD operations
// Data structure
let items = [
{ id: 1, name: "Item 1", description: "Description of Item 1" },
{ id: 2, name: "Item 2", description: "Description of Item 2" },
{ id: 3, name: "Item 3", description: "Description of Item 3" }
];
// Function to add new item
function addItem(name, description) {
let newItem = {
id: items.length + 1,
name: name,
description: description
};
items.push(newItem);
displayItems();
}
// Function to display items
function displayItems() {
let itemListHTML = "";
items.forEach(item => {
itemListHTML += `<div>
<p><strong>${item.name}</strong>: ${item.description}</p>
<button onclick="editItem(${item.id})">Edit</button>
<button onclick="deleteItem(${item.id})">Delete</button>
</div>`;
});
document.getElementById("itemList").innerHTML = itemListHTML;
}
// Function to delete item
function deleteItem(id) {
items = items.filter(item => item.id !== id);
displayItems();
}
// Function to edit item
function editItem(id) {
const itemToEdit = items.find(item => item.id === id);
const newName = prompt("Enter new name:", itemToEdit.name);
const newDescription = prompt("Enter new description:", itemToEdit.description);
if (newName !== null && newDescription !== null) {
itemToEdit.name = newName;
itemToEdit.description = newDescription;
displayItems();
}
}
// Initial display of items
displayItems();
// Add event listener for form submission
document.getElementById("addItemForm").addEventListener("submit", function(event) {
event.preventDefault(); // Prevent default form submission
const name = document.getElementById("itemName").value;
const description = document.getElementById("itemDescription").value;
addItem(name, description);
});
Now let’s deep dive in each Javascript CRUD Operations aspect :
Creating Data Structures To demonstrate CRUD operations, let’s define a simple data structure representing a collection of items. Each item will have an ID, a name, and a description, stored in an array.
let items = [
{ id: 1, name: "Item 1", description: "Description of Item 1" },
{ id: 2, name: "Item 2", description: "Description of Item 2" },
{ id: 3, name: "Item 3", description: "Description of Item 3" }
];
Performing JavaScript CRUD Operations Now, let’s dive into implementing each CRUD operation:
- Create Operation: Adding New Items We’ll create a form to allow users to add new items. Upon form submission, the new item will be added to the collection.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>JavaScript CRUD Example</title>
</head>
<body>
<h1>JavaScript CRUD Example</h1>
<form id="addItemForm">
<label for="itemName">Name:</label>
<input type="text" id="itemName" required>
<label for="itemDescription">Description:</label>
<input type="text" id="itemDescription" required>
<button type="submit">Add Item</button>
</form>
<div id="itemList">
<!-- Existing items will be displayed here -->
</div>
<script src="crud.js"></script>
</body>
</html>
Also look at the Code is Visual Studio
This is how the code is structured in Visual Studio,Below is the screenshot for html file
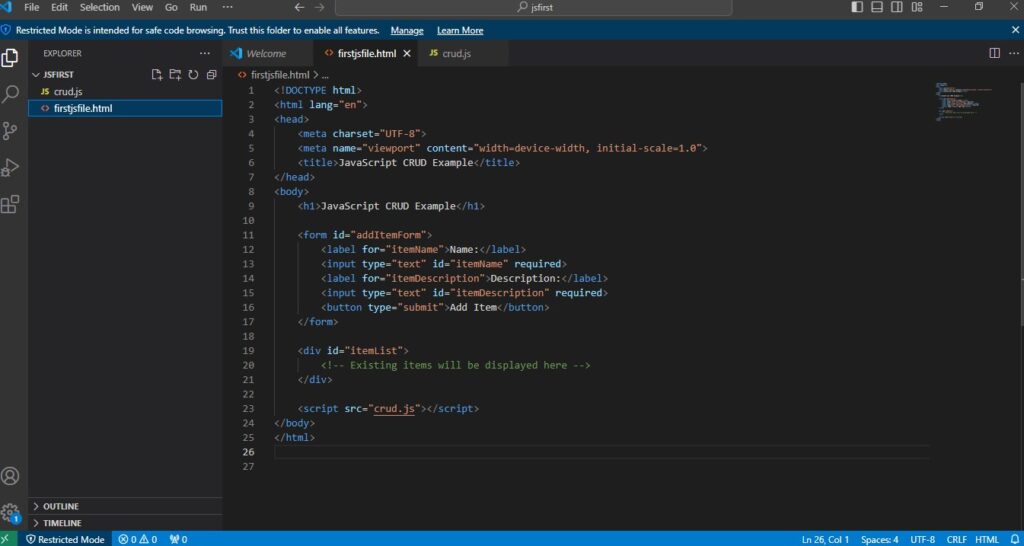
Here is the screenshot for javascript(CRUD) file
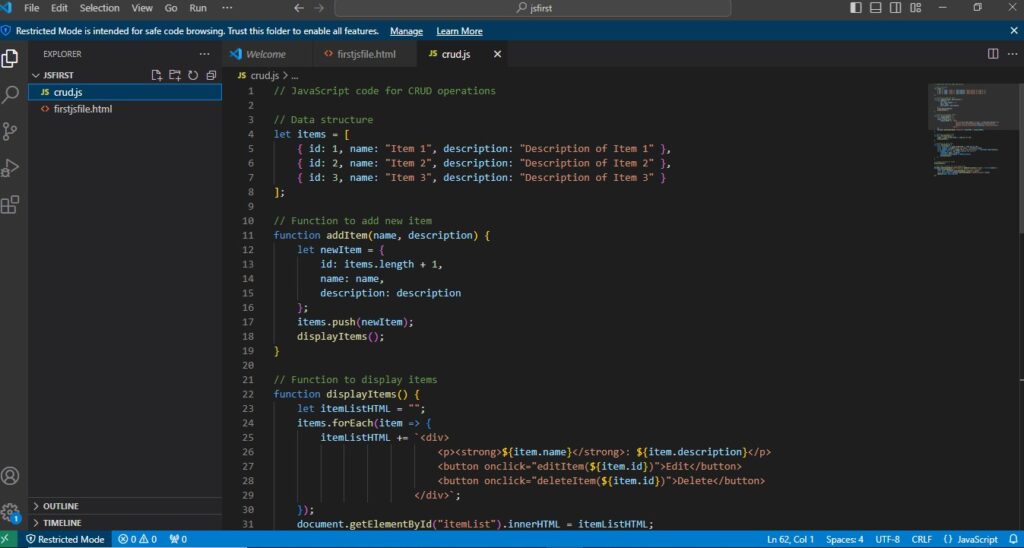
Running the html in a browser for javascript crud operations
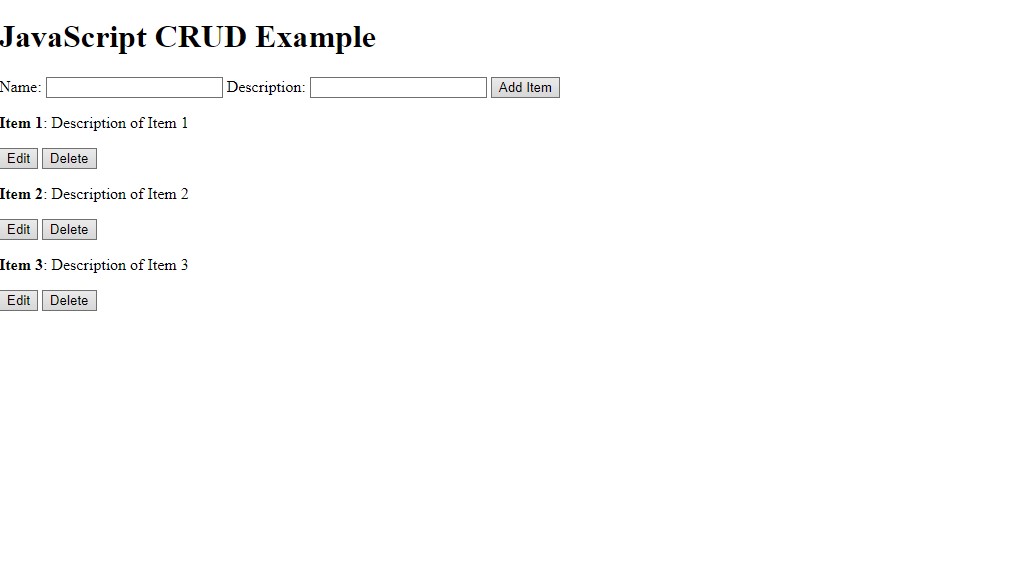
Entering date in html fields
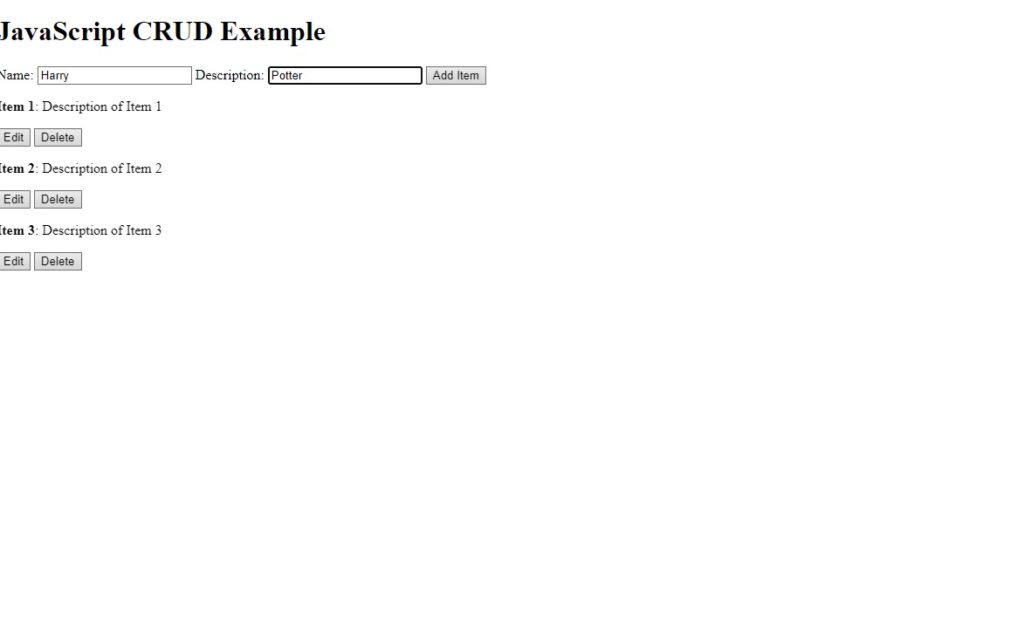
You can see fields are added in the page.
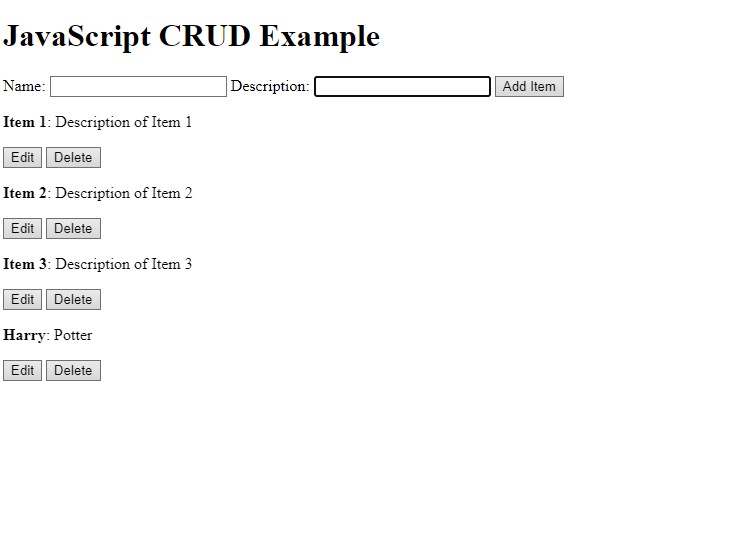
Happy Coding.
Also learn more on